0. 사전에 준비해야 할것
0-1. 사용하고자하는 이메일에서 smtp가 가능한지 확인
0-2. 만약 가능하다면 smtp의 주소와 포트 번호를 확인한다.
1. NuGet 에서 MimeKit 을 다운로드 받습니다.
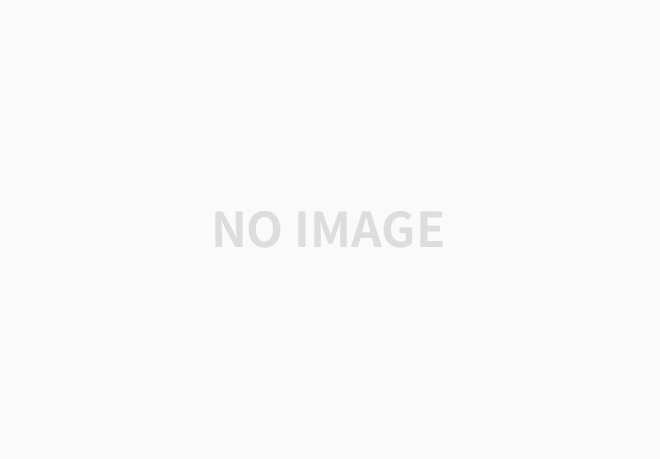
2. MailSender.cs 를 원하는 폴더에 구현합니다.
using MimeKit;
using System;
using System.IO;
using System.Text;
namespace Project.Common
{
public class MailSender
{
// toMail: 보내고자하는 이메일주소
// title: 보내고자하는 이메일의 제목
// mailContent : 보내고자하는 이메일의 내용(HTML 태그형식의 string 값)
public void Send(string toMail, string title, string mailContent ) {
if (toMail.IndexOf("@") > -1)
{
string id = (toMail.Split('@')[0]).ToString();
using (var client = new MailKit.Net.Smtp.SmtpClient())
{
// 사용하고자하는 email의 smtps 주소, 포트를 입력합니다.
client.Connect("smtps.SMTPS_ADDRESS.com", SMTPS_PORT, true);
// 당신의 아이디와 비밀번호를 입력합니다.
client.Authenticate("YOUR_ID", "YOUR_PASSWORD");
var message = new MimeMessage();
//받는사람이 보는
// 보내는 사람의 대표이름과 보내는 사람 이메일을 설정합니다.
message.From.Add(new MailboxAddress("EMAIL_TITLE", "EMAIL_FROM"));
message.To.Add(new MailboxAddress(id, toMail));
message.Subject = title;
var builder = new BodyBuilder();
builder.HtmlBody = mailContent;
message.Body = builder.ToMessageBody();
client.Send(message);
client.Disconnect(true);
}
}
}
}
}
어디서든지 Send를 통해 보내면됩니다.
'ASP.NET > .NET Framework' 카테고리의 다른 글
asp.net mvc 다중 이미지 업로드 (0) | 2020.05.13 |
---|---|
Upload Files In ASP.NET MVC 5 (0) | 2020.05.12 |
ASP.NET MVC JsonResult Date Format / asp.net json date 값 받기 (0) | 2020.05.12 |
asp.net MVC4 파일/이미지 업로드 ( Uploading files in ASP.NET MVC4 ) (0) | 2020.05.06 |
ASP.NET 한글 깨짐 현상 인코딩 해결방법 (0) | 2020.04.28 |